Microsoft Dynamics 365 Business Central Developer (beta) MB-820 Dumps Questions and Answers
Exam Code:
MB-820
Exam Name:
Microsoft Dynamics 365 Business Central Developer (beta)
Certification:
Microsoft Certified - Dynamics 365 Business Central Developer Associate Certification
Vendor:
Microsoft
Exam Questions:
56
Last Updated:
Apr 29, 2024
Exam Status:
Stable
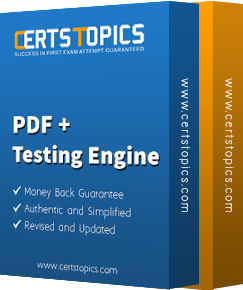
Microsoft Microsoft Certified - Dynamics 365 Business Central Developer Associate Certification MB-820 Exam Dumps
CertsTopics offers Microsoft Microsoft Dynamics 365 Business Central Developer (beta) real exam questions and practice test engine with real questions and verified answers. Try Microsoft Dynamics 365 Business Central Developer (beta) exam questions for free. You can also download a free PDF demo of Microsoft Microsoft Dynamics 365 Business Central Developer (beta) exam. Our Microsoft Dynamics 365 Business Central Developer (beta) Combo Package for which includes PDF (Printable Format) and Testing Engine (Works on Windows and MAC) which ensures you to go through all certification topics and provides you ultimate satisfaction to pass your exam in your first attempt.