Cloudera CCA175 Exam With Confidence Using Practice Dumps
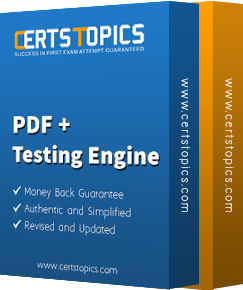
CCA175: Cloudera Certified Associate CCA Exam 2025 Study Guide Pdf and Test Engine
Are you worried about passing the Cloudera CCA175 (CCA Spark and Hadoop Developer Exam - Performance Based Scenarios) exam? Download the most recent Cloudera CCA175 braindumps with answers that are 100% real. After downloading the Cloudera CCA175 exam dumps training , you can receive 99 days of free updates, making this website one of the best options to save additional money. In order to help you prepare for the Cloudera CCA175 exam questions and verified answers by IT certified experts, CertsTopics has put together a complete collection of dumps questions and answers. To help you prepare and pass the Cloudera CCA175 exam on your first attempt, we have compiled actual exam questions and their answers.
Our (CCA Spark and Hadoop Developer Exam - Performance Based Scenarios) Study Materials are designed to meet the needs of thousands of candidates globally. A free sample of the CompTIA CCA175 test is available at CertsTopics. Before purchasing it, you can also see the Cloudera CCA175 practice exam demo.